How the web works If you’re new to web development, you might think you have a basic understanding of how the web operates. But when it comes to explaining it step by step, things can get a little fuzzy.
What exactly is an IP address? How does the client-server model function?
With modern development frameworks handling much of the complexity, it’s easy to overlook the fundamentals. But the deeper you dive—especially into backend development—the more you realize just how crucial these basics are.
That’s why I’ve put together this four-part field guide to help demystify the key concepts every web developer should know:
- Part 1: How the Web Works
- Part 2: Structure of a Web Application
- Part 3: HTTP and REST
- Part 4: Code Examples of Client-Server Interactions
A Simple Web Search
Let’s start with something familiar: typing www.github.com
into your browser. The page loads seamlessly, but under the hood, a series of intricate processes take place.
Breaking Down the Web’s Core Components
To understand how this works, we need to define some key terms:
- Client: A device (like your browser) that interacts with the web. It sends requests to servers and displays the responses.
- Server: A computer that stores and serves web pages upon request.
- IP Address: A unique identifier for devices on a network, allowing them to communicate.
- ISP (Internet Service Provider): The intermediary that connects your device to the internet and resolves domain names into IP addresses.
- DNS (Domain Name System): A system that maps domain names (e.g.,
github.com
) to their corresponding IP addresses. - HTTP (Hypertext Transfer Protocol): The communication protocol used between web browsers and servers.
- URL (Uniform Resource Locator): The address used to locate a specific resource on the web.
The Journey from URL to Webpage

Here’s what happens when you type www.github.com
into your browser:
- The browser interprets the URL and identifies the protocol (
https
), domain name (github.com
), and requested resource. - It contacts the ISP, which performs a DNS lookup to find the IP address of the server hosting
github.com
. - Once the IP address is found, the browser opens a connection to the server.
- The browser sends an HTTP request for the webpage.
- The server processes the request and responds with the HTML page.
- The browser retrieves additional resources (CSS, JavaScript, images) to render the page fully.
- The web page is displayed in the browser.
How the web works
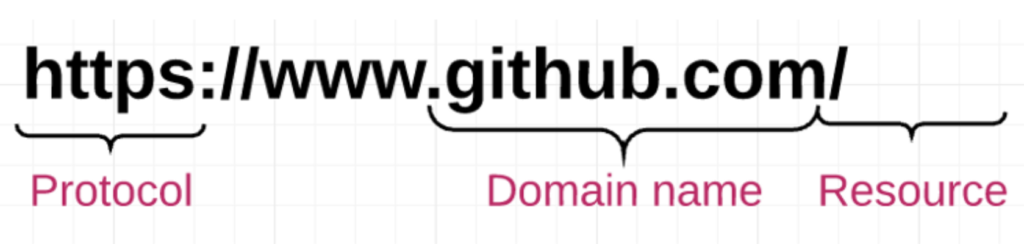
How Data Travels Across the Internet
When you request a webpage, the information is broken into packets that travel independently across the internet. These packets:
- Contain source and destination IP addresses.
- Are transmitted through various network routes.
- Are reassembled by the receiving device using TCP/IP protocols.
Rendering the Webpage
Once all the necessary data arrives, the browser:
- Parses HTML to construct the DOM (Document Object Model).
- Processes CSS to determine how elements should be styled.
- Executes JavaScript to add interactivity.
- Renders the final webpage on your screen.
A Simple Web Search
Breaking Down the Web’s Core Components
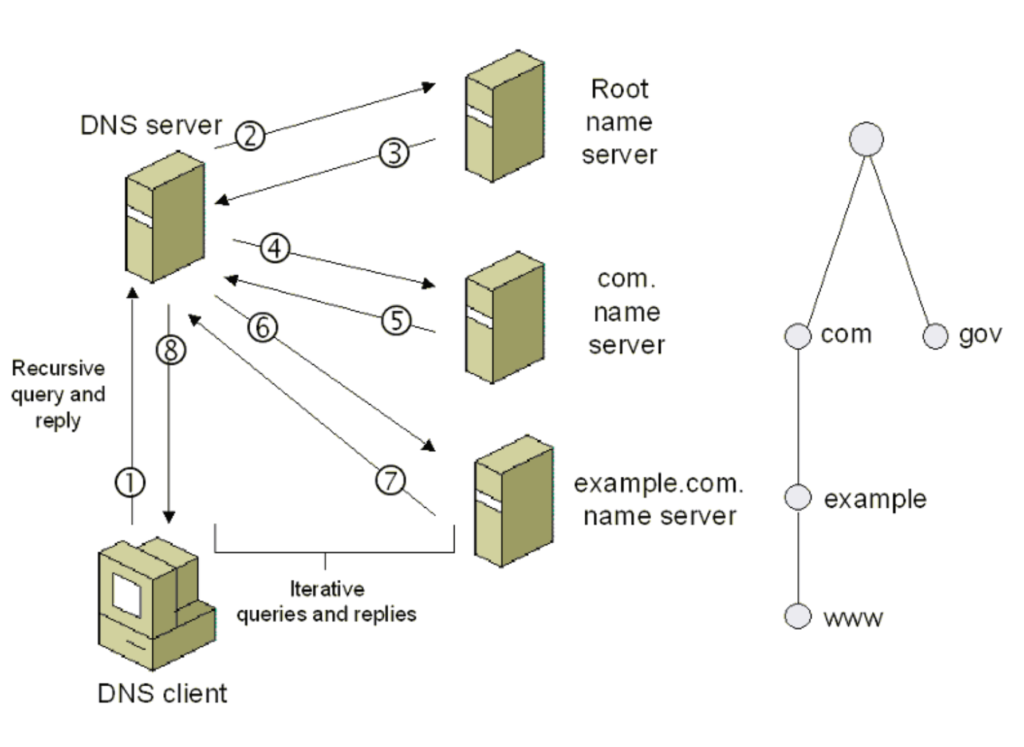
To fully understand how the web functions, we first need to define its key components.
Client: The Starting Point of Any Web Request
A client is any device (laptop, phone, tablet) that can request information from a server. The most common client application is a web browser (Chrome, Firefox, Edge, Safari).
When you enter a website URL, your browser acts as a mediator, translating your actions into HTTP requests sent to web servers.
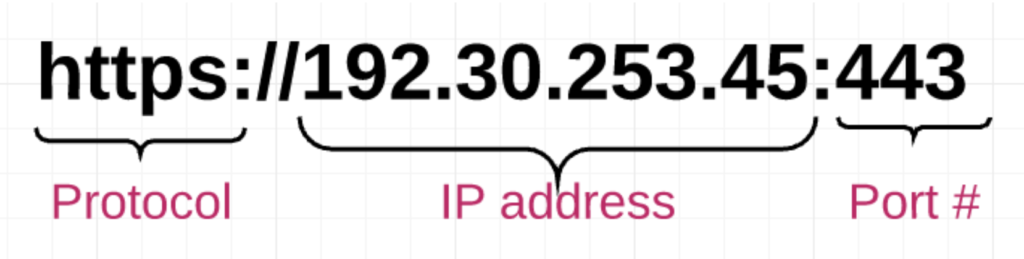
The client is responsible for:
- Taking user input (like typing a URL or clicking a link).
- Sending requests to web servers over the internet.
- Displaying the response received from the server (HTML, images, CSS, JavaScript).
Server: The Machine That Hosts and Delivers Webpages
A server is a specialized computer designed to store, process, and serve web content. Unlike your personal computer, which might be turned off or disconnected, web servers are:
- Always online and connected to the internet.
- Running software (Apache, Nginx, IIS) that knows how to handle HTTP requests.
- Serving multiple clients at once.
A server listens for incoming requests, retrieves the requested resource (HTML, database records, images), and sends the appropriate response back to the client.
IP Address: The Internet’s Unique Identification System
Every device on the internet has a unique identifier called an IP address. This address allows different machines to locate and communicate with each other.
There are two types of IP addresses:
- IPv4: A 32-bit format (
192.168.1.1
) with a limited number of unique addresses. - IPv6: A 128-bit format (
2001:0db8:85a3::7334
), designed to accommodate the growing number of internet-connected devices.
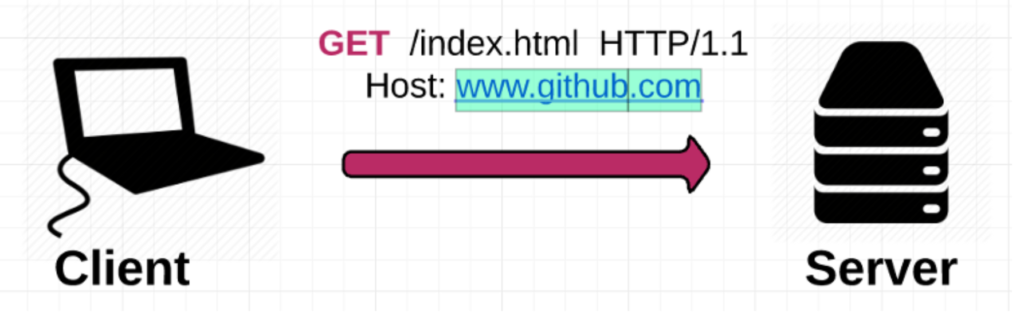
ISP: The Middleman Between You and the Internet
Your Internet Service Provider (ISP) is the company that connects you to the internet. It acts as an intermediary between your client device and the web server you are trying to reach.
When you enter a website address, your ISP:
- Performs a DNS lookup to find the correct IP address.
- Routes your request to the destination server.
- Ensures data packets are transmitted correctly between your browser and the website.
DNS: Translating Domain Names to IP Addresses
The Domain Name System (DNS) is like a phonebook for the internet. Instead of memorizing complex numerical IP addresses, we use domain names like www.github.com
.
When you enter a domain name:
- Your browser asks the DNS server for the corresponding IP address.
- The DNS server looks up the address and returns it to the browser.
- The browser uses this IP address to connect to the correct web server.
Without DNS, you would need to type IP addresses directly, making the web much harder to navigate.
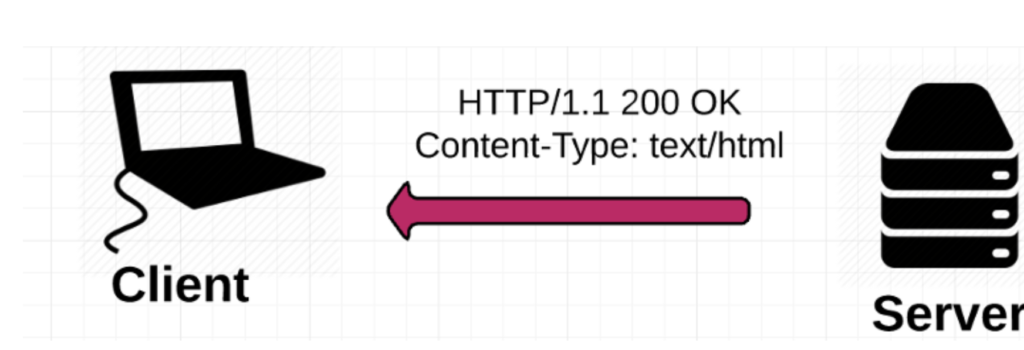
HTTP: The Language of the Web
Hypertext Transfer Protocol (HTTP) is the set of rules that browsers and servers use to communicate. It defines how requests and responses are formatted.
- HTTP (port 80): Standard communication protocol.
- HTTPS (port 443): A secure version of HTTP that encrypts data using SSL/TLS.
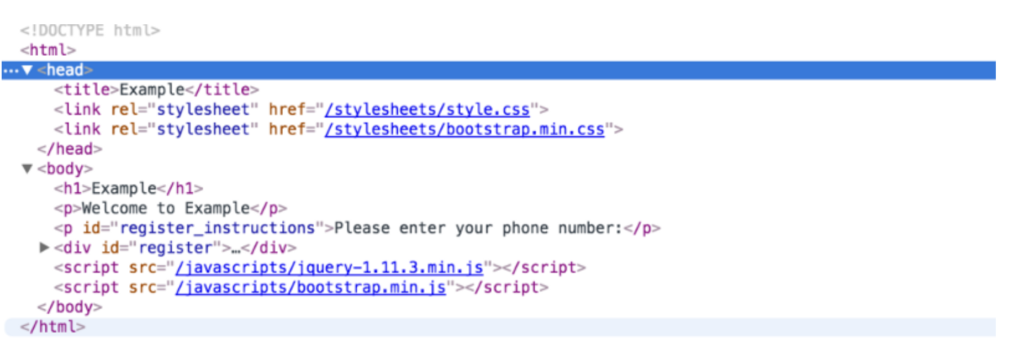
Every time you visit a website, your browser and the web server exchange HTTP messages to transfer the necessary files.
URL: The Address of a Web Resource
A Uniform Resource Locator (URL) is the complete address that identifies a specific web resource.
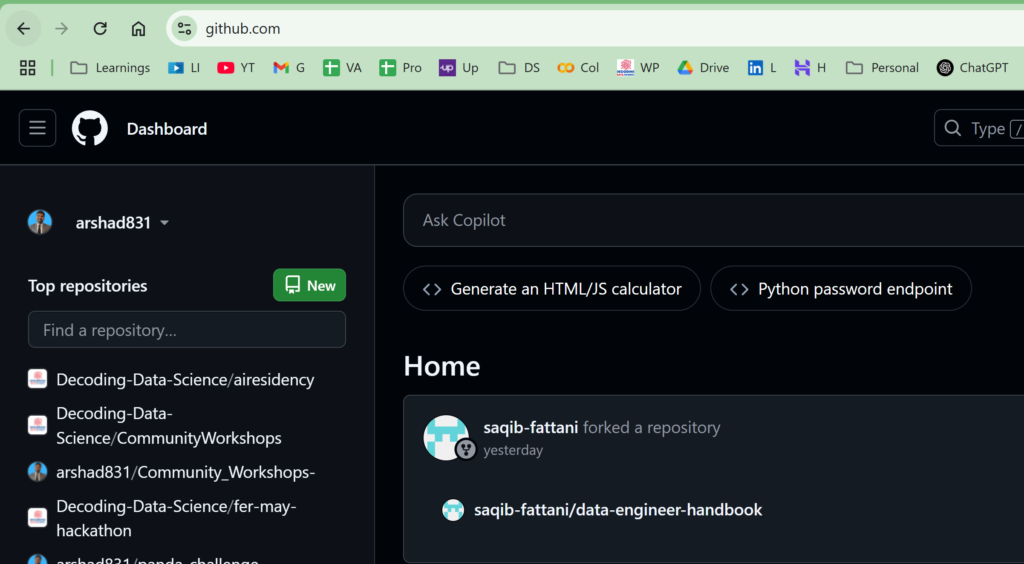
https://github.com/user/profile
A URL consists of:
- Protocol (
https://
) – Specifies how data is transmitted. - Domain (
github.com
) – The address of the web server. - Path (
/user/profile
) – The specific resource being requested.
The Journey from URL to Webpage: A Step-by-Step Breakdown
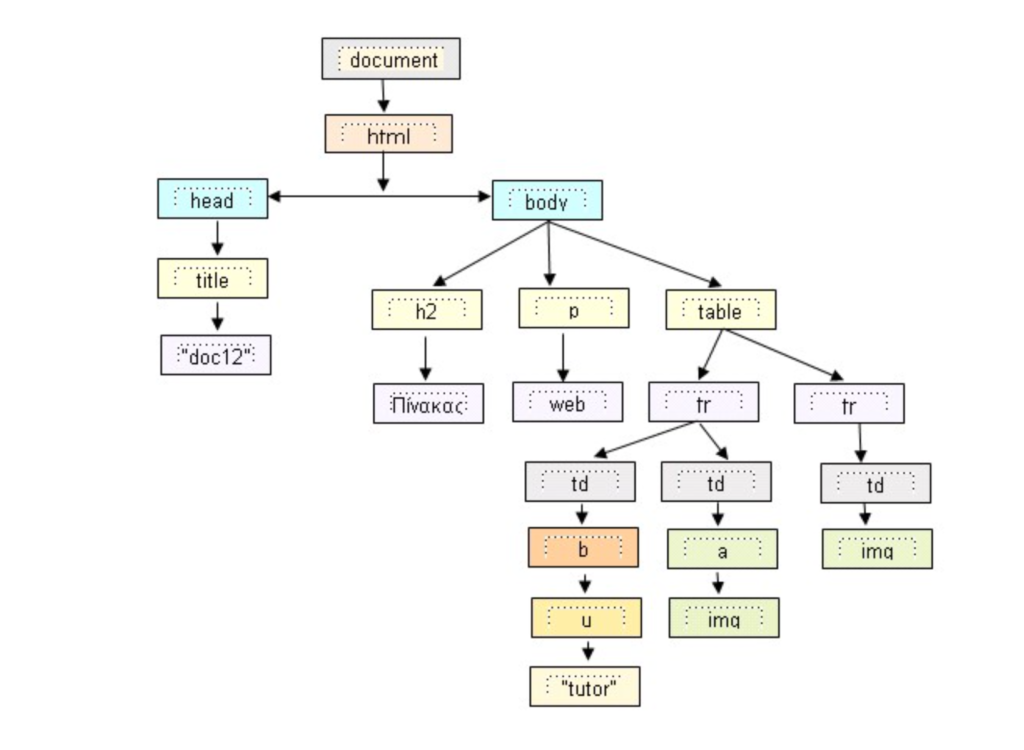
Let’s revisit the scenario where you type www.github.com
into your browser and analyze each stage of the process.
- You type a URL into the browser
- The browser interprets the URL structure and identifies the domain name.
- The browser contacts your ISP for a DNS lookup
- The ISP queries a DNS server to resolve
github.com
into an IP address.
- The ISP queries a DNS server to resolve
- The browser establishes a connection to the web server
- Using the retrieved IP address, the browser initiates a TCP connection with the server.
- An HTTP request is sent to the server
- The browser makes a GET request for the web page.
- The web server processes the request
- If the requested page exists, the server sends the HTML response.
- If not, it sends a
404 Not Found
error.
- The browser parses the response
- The browser reads the HTML and requests additional resources (CSS, JavaScript).
- The browser loads the webpage and renders it
- It constructs the Document Object Model (DOM) and applies styles.
- The webpage is displayed
- The user finally sees the fully rendered page.
Mastering the Web’s Foundations
The web is a complex but well-structured system. Understanding these fundamentals will:
- Help you troubleshoot web-related issues.
- Improve your ability to build web applications.
- Give you a deeper appreciation for how the internet works.
In the next parts of this series, we’ll explore web application structure, HTTP and REST, and client-server interactions with code examples.
If you’re looking to jumpstart your career as a Data Scientist, consider enrolling in our comprehensive AI Residency Program Our program provides you with the skills and experience necessary to succeed in today’s data-driven world. You’ll learn the fundamentals of statistical analysis, as well as how to use tools such as SQL, Python, Excel, and PowerBI to analyze and visualize data designed by Mohammad Arshad, 19 years of Data Science & AI Experience. But that’s not all – our program also includes a 3-month internship with us where you can showcase your Capstone Project.
Are you passionate about AI and Data Science? Looking to connect with like-minded individuals, learn new concepts, and apply them in real-world situations? Join our growing AI community today! We provide a platform where you can engage in insightful discussions, share resources, collaborate on projects, and learn from experts in the field.
Don’t miss out on this opportunity to broaden your horizons and sharpen your skills. Visit https://decodingdatascience.com/ai and be part of our AI community. We can’t wait to see what you’ll bring to the table. Let’s shape the future of AI together!