In the era of data-driven decision-making, the ability to visualize data effectively has become a critical skill. Among the many tools available, Matplotlib, a versatile Python library, stands out as one of the most popular choices for creating compelling visualizations. Whether you’re a data scientist, analyst, or researcher, mastering Matplotlib can elevate your data storytelling capabilities. This guide dives deep into the essentials of Matplotlib, its features, and how you can leverage it to create impactful visualizations.Matplotlib tutorial
What is Matplotlib?
Matplotlib is a Python 2D plotting library that enables users to generate static, interactive, and animated visualizations. Created by John D. Hunter in 2003, it has become a cornerstone in the Python data visualization ecosystem. It integrates seamlessly with NumPy, Pandas, and other Python libraries, making it a preferred choice for developers and data professionals.
Key Features of Matplotlib
- Wide Range of Plot Types:
- Line plots, bar charts, histograms, scatter plots, and more.
- Customizability:
- Fine-tune every aspect of your plot, from colors and fonts to markers and gridlines.
- Integration with Other Libraries:
- Works well with NumPy, Pandas, and SciPy for seamless data manipulation and visualization.
- Interactive Plots:
- Supports backends like Tkinter, Qt, and Jupyter Notebooks for interactive visualizations.
- Publication-Quality Figures:
- Create high-resolution plots suitable for reports and academic papers.
Getting Started with Matplotlib
Installation
To install Matplotlib, you can use pip:
pip install matplotlib
Alternatively, for Anaconda users:
conda install matplotlib
Basic Usage
To begin, import the library:
import matplotlib.pyplot as plt
Creating Your First Plot
Here’s a simple example of a line plot: Matplotlib tutorial
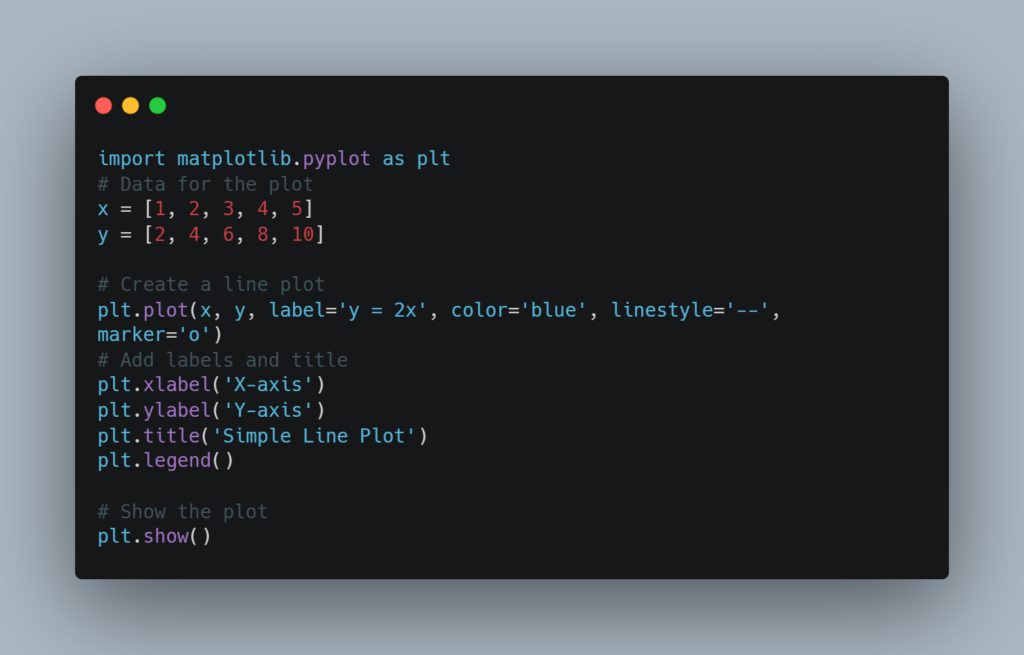
This code generates a simple line graph with labeled axes, a title, and a legend.
Advanced Plot Types
1. Scatter Plots
Scatter plots are ideal for visualizing relationships between two variables.
import matplotlib.pyplot as plt
import numpy as np
# Generate random data
x = np.random.rand(50)
y = np.random.rand(50)
sizes = np.random.randint(20, 200, 50)
colors = np.random.rand(50)
# Create scatter plot
plt.scatter(x, y, s=sizes, c=colors, alpha=0.7, cmap='viridis')
plt.colorbar(label='Color Intensity')
plt.title('Scatter Plot Example')
plt.show()
2. Bar Charts
Bar charts are useful for comparing categorical data.
categories = ['A', 'B', 'C', 'D']
values = [10, 15, 7, 10]
plt.bar(categories, values, color='skyblue')
plt.title('Bar Chart Example')
plt.xlabel('Categories')
plt.ylabel('Values')
plt.show()
3. Histograms
Histograms display the distribution of a dataset.
import numpy as np
# Generate random data
data = np.random.randn(1000)
plt.hist(data, bins=30, color='purple', edgecolor='black', alpha=0.7)
plt.title('Histogram Example')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.show()
4. Pie Charts
Pie charts represent proportions within a dataset.
labels = ['Python', 'Java', 'C++', 'JavaScript']
sizes = [40, 25, 20, 15]
colors = ['gold', 'lightcoral', 'lightskyblue', 'yellowgreen']
explode = (0.1, 0, 0, 0) # Explode the first slice
plt.pie(sizes, explode=explode, labels=labels, colors=colors, autopct='%1.1f%%', shadow=True, startangle=140)
plt.title('Programming Language Usage')
plt.show()
Customizing Matplotlib Plots
1. Adding Gridlines
plt.plot([1, 2, 3], [4, 5, 6])
plt.grid(True, linestyle='--', linewidth=0.5, alpha=0.7)
plt.show()
2. Adjusting Figure Size and DPI
plt.figure(figsize=(10, 6), dpi=100)
plt.plot([1, 2, 3], [4, 5, 6])
plt.show()
3. Annotating Points
x = [1, 2, 3]
y = [4, 5, 6]
plt.plot(x, y, marker='o')
for i in range(len(x)):
plt.text(x[i], y[i], f'({x[i]}, {y[i]})', fontsize=10, ha='right')
plt.show()
4. Changing Themes with Style Sheets
Matplotlib includes various pre-built styles.
import matplotlib.pyplot as plt
plt.style.use('seaborn-darkgrid')
plt.plot([1, 2, 3], [4, 5, 6])
plt.show()
To view available styles:
print(plt.style.available)
Best Practices for Using Matplotlib
- Plan Your Visualizations:
- Determine the purpose of your visualization before coding.
- Keep It Simple:
- Avoid clutter by limiting the number of visual elements.
- Use Consistent Scales:
- Maintain uniform scales across related plots for better comparison.
- Label Everything:
- Always include titles, labels, and legends to enhance readability.
- Exporting Your Plots:
- Save your plots in high resolution for reports or presentations:
plt.savefig('plot.png', dpi=300, bbox_inches='tight')
Matplotlib vs. Other Visualization Libraries
Matplotlib vs. Seaborn:
- Matplotlib:
- Highly customizable.
- More coding required for complex plots.
- Seaborn:
- Built on top of Matplotlib.
- Easier to create statistical plots.
Matplotlib vs. Plotly:
- Matplotlib:
- Great for static plots.
- Plotly:
- Interactive visualizations.
Matplotlib vs. Tableau:
- Matplotlib:
- Ideal for developers and researchers.
- Tableau:
- User-friendly GUI for business analysts.
Conclusion
Matplotlib remains a cornerstone of data visualization in Python. Its flexibility and robust features make it an indispensable tool for anyone working with data. Whether you’re a beginner creating your first line plot or an expert designing publication-ready figures, Matplotlib has you covered. By mastering this library, you can unlock new possibilities for presenting your data in clear and impactful ways.
Start exploring Matplotlib today and transform your data into stories that matter!
If you’re looking to jumpstart your career as a Data Scientist, consider enrolling in our comprehensive AI Residency Program Our program provides you with the skills and experience necessary to succeed in today’s data-driven world. You’ll learn the fundamentals of statistical analysis, as well as how to use tools such as SQL, Python, Excel, and PowerBI to analyze and visualize data designed by Mohammad Arshad, 19 years of Data Science & AI Experience. But that’s not all – our program also includes a 3-month internship with us where you can showcase your Capstone Project.
Are you passionate about AI and Data Science? Looking to connect with like-minded individuals, learn new concepts, and apply them in real-world situations? Join our growing AI community today! We provide a platform where you can engage in insightful discussions, share resources, collaborate on projects, and learn from experts in the field.
Don’t miss out on this opportunity to broaden your horizons and sharpen your skills. Visit https://decodingdatascience.com/ai and be part of our AI community. We can’t wait to see what you’ll bring to the table. Let’s shape the future of AI together!
Matplotlib is a must-have tool for anyone working with data! 📊🔥 Its flexibility and power make it perfect for crafting insightful visualizations. Mastering it can truly enhance data storytelling and decision-making. Excited to explore more!